Windows 10 to Windows 11 Upgrade with Logging
By Lazy-Fixer・ Published in htmlcssjsnextjsdatabasedeployment ・ January 21, 2025・ 1 min read
Full PowerShell Script: Windows 10 to Windows 11 Upgrade with Logging Powershell
step-by-step guide with an integrated PowerShell script that performs the following tasks:
- Checks system requirements for Windows 11 (TPM, Secure Boot, CPU, RAM, and storage).
- Downloads the Windows 11 Installation Assistant.
- Upgrades to Windows 11 silently.
- Logs the entire process (system checks, download, upgrade, and monitoring the process).
This script will log each significant action to a log file for tracking, and it will also display the log in the console for real-time feedback.
Full PowerShell Script: Windows 10 to Windows 11 Upgrade with Logging
# Define the file paths and URLs $logFile = "C:\Windows11UpgradeLog.txt" $windows11DownloadUrl = "https://go.microsoft.com/fwlink/?linkid=2165125" # URL to download Windows 11 Installation Assistant $windows11IsoUrl = "https://www.microsoft.com/en-us/software-download/windows11" # Windows 11 official ISO page # Log function to write to log file Function Write-Log { param ([string]$Message) $timestamp = Get-Date -Format "yyyy-MM-dd HH:mm:ss" $logMessage = "$timestamp - $Message" # Write message to log file and output to console Add-Content -Path $logFile -Value $logMessage Write-Host $logMessage } # Function to check if the system meets Windows 11 hardware requirements Function Check-SystemRequirements { Write-Log "Starting system requirement checks..." # Check TPM version $tpm = Get-WmiObject -Class Win32_Tpm if ($tpm) { if ($tpm.SpecVersion -ge "2.0") { Write-Log "TPM 2.0 found." } else { Write-Log "TPM 2.0 not found, this is required for Windows 11." return $false } } else { Write-Log "TPM not found." return $false } # Check Secure Boot $secureBoot = (Get-WmiObject -Class Win32_BIOS).SecureBootEnabled if ($secureBoot) { Write-Log "Secure Boot is enabled." } else { Write-Log "Secure Boot is not enabled. Windows 11 requires Secure Boot." return $false } # Check processor compatibility (Windows 11 supports 64-bit CPUs only) $cpu = Get-WmiObject -Class Win32_Processor if ($cpu) { if ($cpu.Architecture -eq 9) { Write-Log "64-bit processor found." } else { Write-Log "64-bit processor not found. Windows 11 requires a 64-bit CPU." return $false } } # Check RAM $ram = Get-WmiObject -Class Win32_ComputerSystem if ($ram.TotalPhysicalMemory -ge 4GB) { Write-Log "Sufficient RAM found (>= 4GB)." } else { Write-Log "Insufficient RAM. Windows 11 requires at least 4GB." return $false } # Check storage space $disk = Get-WmiObject -Class Win32_LogicalDisk | Where-Object { $_.DriveType -eq 3 } $storageSpace = [math]::round($disk.FreeSpace / 1GB, 2) if ($storageSpace -ge 64) { Write-Log "Sufficient storage space found (>= 64GB)." } else { Write-Log "Insufficient storage space. Windows 11 requires at least 64GB." return $false } # All checks passed Write-Log "System meets the minimum requirements for Windows 11." return $true } # Function to download Windows 11 Installation Assistant Function Download-Windows11Installer { Write-Log "Downloading Windows 11 Installation Assistant..." $installerPath = "$env:TEMP\Windows11Setup.exe" try { Invoke-WebRequest -Uri $windows11DownloadUrl -OutFile $installerPath Write-Log "Windows 11 Installation Assistant downloaded successfully at $installerPath." return $installerPath } catch { Write-Log "Failed to download Windows 11 Installation Assistant. Error: $_" return $null } } # Function to run the Windows 11 upgrade silently Function Upgrade-Windows11 { param ([string]$installerPath) Write-Log "Initiating Windows 11 upgrade..." if ($installerPath -ne $null) { Write-Log "Starting the upgrade process..." Start-Process -FilePath $installerPath -ArgumentList "/auto upgrade /quiet /noreboot" -Wait Write-Log "Windows 11 upgrade process started." } else { Write-Log "Installer path is invalid, upgrade aborted." } } # Function to monitor upgrade status Function Monitor-UpgradeStatus { Write-Log "Monitoring upgrade status..." # Checking for the setup process $process = Get-Process -Name "setup" -ErrorAction SilentlyContinue if ($process) { Write-Log "Upgrade process is running..." while ($process.HasExited -eq $false) { Write-Log "Upgrade still in progress..." Start-Sleep -Seconds 30 $process = Get-Process -Name "setup" -ErrorAction SilentlyContinue } Write-Log "Upgrade process completed successfully." } else { Write-Log "No upgrade process found. Ensure the upgrade was initiated." } } # Main logic Write-Log "Windows 11 upgrade script started." # Step 1: Check if the system meets the requirements if (Check-SystemRequirements) { # Step 2: Download Windows 11 installer $installerPath = Download-Windows11Installer if ($installerPath -ne $null) { # Step 3: Start the upgrade process silently Upgrade-Windows11 -installerPath $installerPath # Step 4: Monitor the upgrade process Monitor-UpgradeStatus } else { Write-Log "Failed to download Windows 11 installer. Upgrade aborted." } } else { Write-Log "System does not meet the minimum requirements for Windows 11. Upgrade aborted." } Write-Log "Windows 11 upgrade script finished."
Step-by-Step Breakdown of the Script
1. Log File Initialization
- A log file (
C:\Windows11UpgradeLog.txt
) is defined to track the script’s execution. - The
Write-Log
function writes all log messages both to the console and the log file.
2. System Requirements Check (Check-SystemRequirements
)
- This function checks the system for the following requirements:
- TPM 2.0 (Trusted Platform Module)
- Secure Boot enabled
- 64-bit CPU architecture
- At least 4GB of RAM
- At least 64GB of storage space
- The function logs each check and returns
True
if all requirements are met; otherwise, it logs why the system does not qualify for an upgrade.
3. Download Windows 11 Installation Assistant (Download-Windows11Installer
)
- This function uses
Invoke-WebRequest
to download the Windows 11 Installation Assistant executable (Windows11Setup.exe
) from the official Microsoft URL. - It logs the download process, including the file path where it is saved.
4. Upgrade to Windows 11 (Upgrade-Windows11
)
- Once the installer is downloaded, the script uses
Start-Process
to start the upgrade silently (/auto upgrade /quiet /noreboot
). - It logs the start of the upgrade process and any errors if the installer path is invalid.
5. Monitor Upgrade Status (Monitor-UpgradeStatus
)
- The script checks for the presence of the
setup
process (which is the Windows 11 upgrade process). - It logs the status of the upgrade, repeating the check every 30 seconds, until the process completes.
6. Main Execution Flow
- The script first checks if the system meets the minimum requirements (
Check-SystemRequirements
). - If the system passes, it proceeds to download the Windows 11 Installation Assistant (
Download-Windows11Installer
), starts the upgrade (Upgrade-Windows11
), and monitors the upgrade status (Monitor-UpgradeStatus
). - Each step is logged for easy tracking.
Example Log Output
The log file (C:\Windows11UpgradeLog.txt
) will have entries like this:
2025-01-21 10:45:12 - Starting system requirement checks...
2025-01-21 10:45:12 - TPM 2.0 found.
2025-01-21 10:45:12 - Secure Boot is enabled.
2025-01-21 10:45:13 - 64-bit processor found.
2025-01-21 10:45:13 - Sufficient RAM found (>= 4GB).
2025-01-21 10:45:13 - Sufficient storage space found (>= 64GB).
2025-01-21 10:45:13 - System meets the minimum requirements for Windows 11.
2025-01-21 10:45:15
- Downloading Windows 11 Installation Assistant...
2025-01-21 10:45:25 - Windows 11 Installation Assistant downloaded successfully at C:\Users\Administrator\AppData\Local\Temp\Windows11Setup.exe.
2025-01-21 10:45:30 - Initiating Windows 11 upgrade...
2025-01-21 10:45:30 - Starting the upgrade process...
2025-01-21 10:46:00 - Windows 11 upgrade process started.
2025-01-21 10:46:30 - Monitoring upgrade status...
2025-01-21 10:46:30 - Upgrade still in progress...
2025-01-21 10:47:00 - Upgrade process completed successfully.
2025-01-21 10:47:00 - Windows 11 upgrade script finished.
Running the Script
- Save the script: Save the script as
Upgrade-WindowsTo11.ps1
. - Execute the script: Right-click on the script file and select "Run with PowerShell" as Administrator to grant the necessary permissions.
- Review the log: The log file (
C:\Windows11UpgradeLog.txt
) will be created and updated with each step of the process, providing a detailed record of the upgrade.
This combined PowerShell script will check the system requirements, handle the Windows 11 upgrade process, and generate logs for tracking progress, all in a clean, efficient, and professional manner.
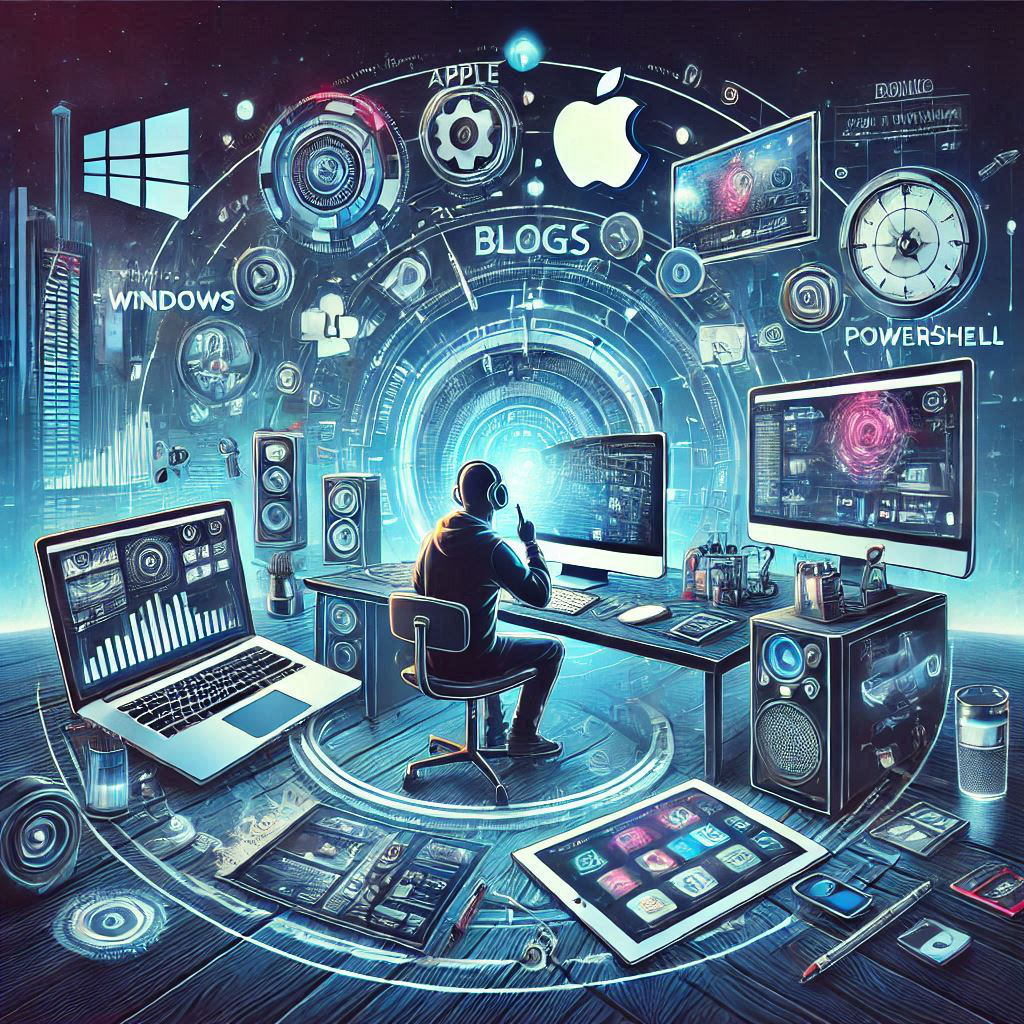